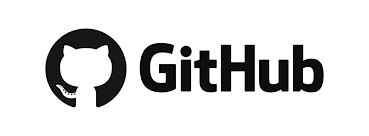
This detailed tutorial will guide you through the full process of using Git with Visual Studio Code (VS Code). From installation to committing changes and managing branches, you'll learn how to effectively use Git without leaving your code editor.
1. Install Git and VS Code
Install Git
- Visit git-scm.com
- Download the correct installer for your operating system (Windows, macOS, or Linux).
- Follow the installation wizard. Keep the default settings unless you have a specific need to change them.
To verify the installation, run the following in your terminal or command prompt:
git --version
[screenshot:image of Git installation verification in terminal]
Install VS Code
- Visit code.visualstudio.com
- Download and install VS Code for your operating system.
[screenshot:image of VS Code homepage or installation window]
2. Set Up Git (First Time Only)
Open your terminal or command prompt and configure Git with your identity:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
These settings are stored in a global config file so that every project uses the same identity.
You can check your config settings with:
git config --list
[screenshot:image of terminal showing git config list output]
3. Clone a Repository
To start working on an existing project, clone its Git repository:
- Copy the repository URL (from GitHub, GitLab, etc.).
- In VS Code, open the Command Palette (
Ctrl+Shift+P
orCmd+Shift+P
on Mac). - Type
Git: Clone
and select it. - Paste the repository URL.
- Choose a folder where the project will be saved locally.
- Once cloned, VS Code will ask if you want to open the folder. Click
Open
.
[screenshot:image of Git clone command in VS Code command palette]
4. Open a Git Repository
If you already have a Git project locally:
- Open VS Code.
- Select
File > Open Folder
and choose your project folder. - VS Code will automatically detect the
.git
folder and enable Git features.
[screenshot:image of VS Code with a Git project opened]
5. Make Changes and Commit
When you edit any tracked file, VS Code will detect the change:
- Click the Source Control icon in the sidebar (
Ctrl+Shift+G
). - All modified files will be listed under "Changes".
- Click a file to view a diff of your changes.
- Type a meaningful commit message in the message box (e.g., "Add feature to handle login").
- Click the check mark (✔) above to commit your changes.
[screenshot:image of VS Code Source Control panel with staged changes]
Tip: Use concise, descriptive commit messages that explain the 'what' and 'why'.
6. Push Changes to Remote
After committing your changes locally, push them to the remote repository:
- Click the three-dot menu (
...
) in the Source Control view. - Choose
Push
. - If prompted to set the upstream branch, confirm the default option.
You can also use the terminal:
git push
[screenshot:image of push button in Source Control menu]
7. Pull Latest Changes
To update your local copy with the latest changes from the remote:
- Open the Command Palette and choose
Git: Pull
. - Or open the Source Control menu (
...
) >Pull
.
In terminal:
git pull
[screenshot:image of pull action in VS Code]
Tip: Always pull before starting work to avoid merge conflicts.
8. Create and Switch Branches
Create a New Branch:
- Click the branch name in the bottom-left status bar.
- Select
Create new branch
. - Enter a name for the branch (e.g.,
feature/navbar
).
[screenshot:image of creating a new branch in VS Code]
Switch Between Branches:
- Click the branch name again and select the branch you want to switch to.
- VS Code will reload the current folder based on the branch.
In terminal:
git checkout -b new-branch-name # create and switch
# or
git checkout existing-branch-name
[screenshot:image of branch switching in VS Code status bar]
9. View History and Diffs
View Commit History:
- Right-click any file and choose
View Git History
(with GitLens or Git History extension). - Use the Source Control panel to see recent commits.
[screenshot:image of GitLens history view]
View Changes:
- Click on a file in the Source Control panel to see the changes side-by-side.
- You can stage individual lines or hunks before committing.
[screenshot:image of file diff in VS Code]
10. Extensions to Enhance Git Experience
GitLens
- Supercharge your Git workflow with authorship info, blame annotations, commit history, and more.
- Install from Extensions panel: search for
GitLens
[screenshot:image of GitLens extension page in VS Code]
Git Graph
- Visualize your commit history and branches in a graph format.
- Install from Extensions panel: search for
Git Graph
[screenshot:image of Git Graph visualization]
11. Useful Git Commands
git status # Check current status
git add . # Stage all changes
git add <file> # Stage specific file
git commit -m "Message" # Commit changes
git push # Push changes to remote
git pull # Pull latest changes
git fetch # Fetch without merge
git checkout -b new-branch # Create and switch to new branch
git checkout main # Switch to main branch
git log # View commit history in terminal
[screenshot:image of terminal using common Git commands]
12. Troubleshooting Tips
- Merge Conflicts:
- When two people edit the same part of a file, Git will flag a conflict.
- VS Code highlights conflicts, and you can choose to accept either side or combine them manually.
[screenshot:image of merge conflict resolution in VS Code]
-
Authentication Issues:
- Make sure you're signed in via the GitHub extension or SSH key is set up.
-
Push Rejected:
- Run
git pull
to update your local changes before pushing again.
- Run
Conclusion
VS Code makes it easy to manage Git repositories without using the command line. Whether you're collaborating on a team or working solo, learning Git will greatly enhance your development workflow.
Happy coding! 🎉