Setup Laravel API: A Complete Guide for Beginners
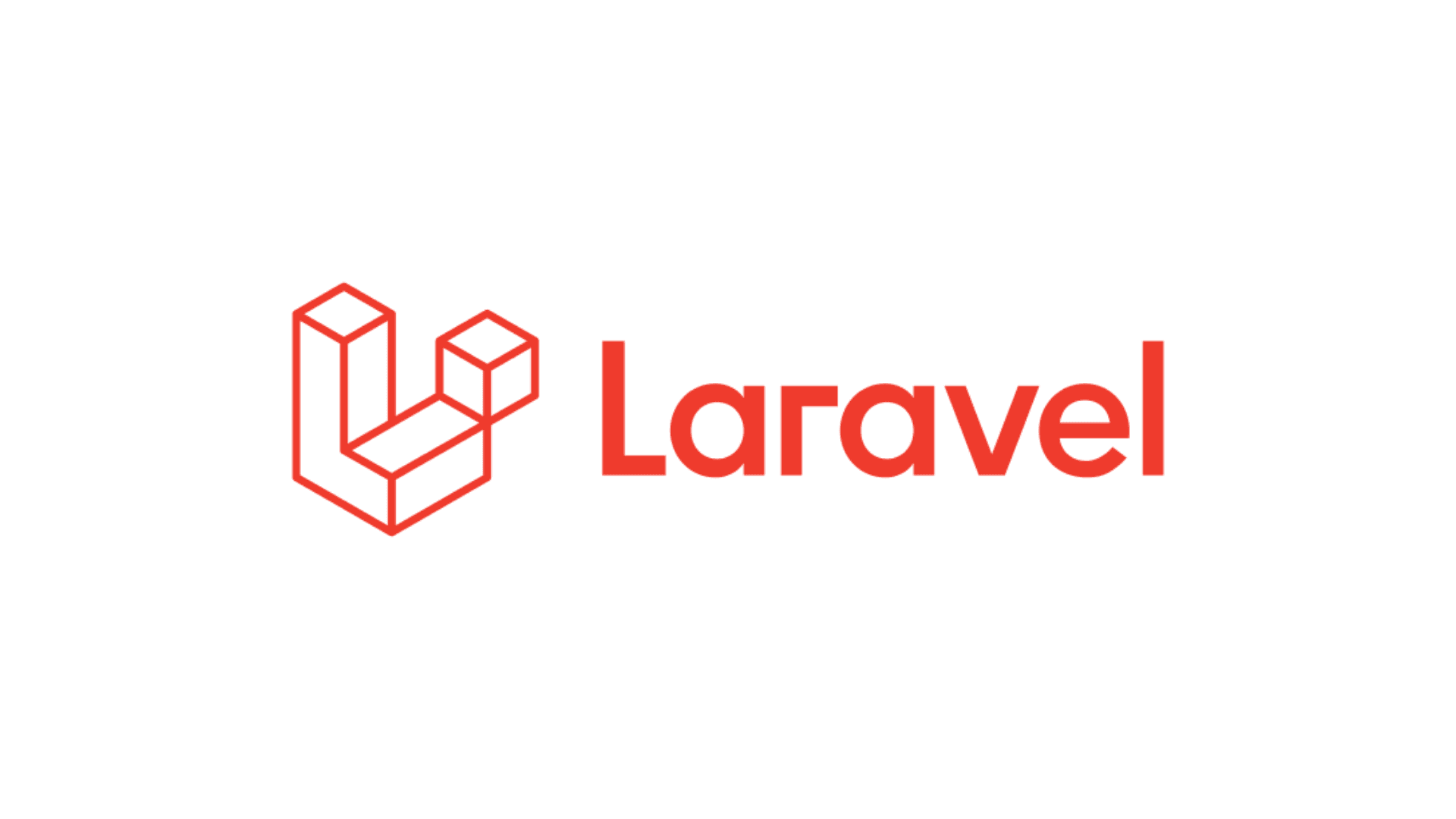
Laravel is one of the most popular PHP frameworks that offers simplicity in building web applications and RESTful APIs. In this guide, we will learn how to set up Laravel specifically for API development.
Prerequisites
Make sure you have installed:
- PHP >= 8.1
- Composer
- A Database (MySQL, PostgreSQL, etc.)
- Laravel Installer (optional)
1. Create a New Laravel Project
Open your terminal and run one of the following commands:
Using Composer:
composer create-project laravel/laravel laravel-api
Or using Laravel Installer:
laravel new laravel-api
Navigate into the project folder:
cd laravel-api
2. Configure the Database
Open the .env
file and adjust your database configuration:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_api
DB_USERNAME=root
DB_PASSWORD=
Then run the default Laravel migrations:
php artisan migrate
3. Create an API Resource
Example: We want to create an API endpoint for Post
.
Run the following command:
php artisan make:model Post -mcr
This command will generate:
Post
model- Migration file
PostController
(resource)- Automatic route in
routes/api.php
Edit the migration file in database/migrations/...create_posts_table.php
:
$table->string('title');
$table->text('content');
Then run the migration:
php artisan migrate
4. Define API Routes
Open the routes/api.php
file:
Route::apiResource('posts', PostController::class);
5. Test API with Postman
Laravel automatically formats responses in JSON. Try accessing these endpoints:
GET /api/posts
POST /api/posts
PUT /api/posts/{id}